Protect your data with AESCryptor in Unreal Engine 5
Leverage AES-128/192/256 encryption through Blueprint nodes or C++ functions. Secure save games, API keys, and more on Windows.
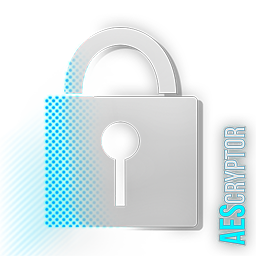
OVerview
The AESCryptor plugin provides powerful AES encryption and decryption capabilities directly in Unreal Engine 5. It supports three key sizes (128-bit, 192-bit, 256-bit) and allows developers to secure their sensitive data using industry-standard encryption directly through Blueprint nodes or C++ functions.
- AES Encryption & Decryption
- Blueprint Integration, use with Blueprints
- C++ Integration, use with CPP
- 128-, 192-, 256-bit Support
- Uses UTF-8
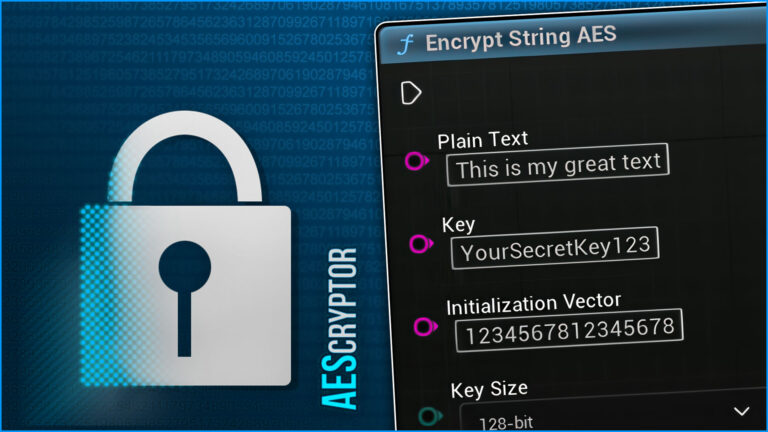
AESCryptor: Showcase
Protect your game saves easily with AESCryptor. Using AES-128/192/256 encryption, secure sensitive data like save games and API keys directly through Blueprint nodes or C++ functions. AESCryptor is perfect for Unreal Engine projects on Windows, offering high security with easy integration. Its broad platform support and detailed documentation make it simple to get started, whether you’re a beginner or a pro.
Documentation & News
Getting Started
- Download the AESCryptor plugin and place it in the
Plugins
folder of your Unreal Engine project. - Navigate to
Edit > Plugins
and enable AESCryptor. - Restart the editor once more to apply the changes.
- Download the AESCryptor plugin from the FAB Marketplace and import it into your Unreal Engine project.
- Navigate to
Edit > Plugins
and enable AESCryptor. - Restart the editor once more to apply the changes.
General Explanation
Encryption Node
Encrypted Text: This is the text, message, or password that you want to secure through encryption. After encryption, this text will be converted into an unreadable format until decrypted.
Key: This is your secret key or password used to encrypt the text. The Key is required to decrypt the encrypted text and recover the original message. This key must remain confidential, as anyone with access to it can decrypt your data.
Initialization Vector (IV): The IV acts as a “second password” that adds randomness to your encryption, ensuring that even identical text inputs result in different encrypted outputs. The IV should be randomly generated each time you encrypt new data. Unlike the Key, the IV does not need to be kept secret and can be shared along with the encrypted text. It prevents repetition patterns, making encryption more secure.
- Example: If you encrypt the phrase “Test” using the same key each time, the encrypted result will always look the same. However, by using a randomly generated IV for each encryption, the resulting encrypted text will be different every time, even if the input phrase and key remain the same.
Key Size: This defines the strength of the encryption. You can choose between 128-bit, 192-bit, and 256-bit encryption levels:
- 128-bit: Requires a 16-character key. Faster but less secure than 256-bit.
- 192-bit: Requires a 24-character key. Balances security and performance.
- 256-bit: Requires a 32-character key. This provides the highest level of security but may be slower in performance. Recommended for scenarios where maximum data protection is required.
- Note: The key length (in characters) must match the chosen key size for AES encryption to work properly.
Return: You will get the encrypted not-readable Text as String.
Decryption Node
Encrypted Text: This is the text that has already been encrypted and is unreadable in its current form. The Decrypt String AES node will convert this unreadable text back into clear, original text.
Key: This is your secret key or password used for both encryption and decryption. The Sender and Receiver must both know this key to decrypt the message and make the text readable again.
Initialization Vector (IV): Initialization Vector (IV): The IV acts as a “second password” that adds randomness to your encryption, ensuring that even identical text inputs produce different encrypted outputs. For decryption, you need the exact same IV that was used during encryption to properly decrypt the text. The IV can safely be shared along with the encrypted text.
- Example: In this example, I appended the IV to the Encrypted String using an Append Node. Now, I use the “Parse Into Array” node to split the Encrypted Text from the included IV, making it easier to handle both components separately.
Key Size: This defines the strength of the encryption. The decryption key size must match the encryption key size. For example, 128-bit encryption requires 128-bit decryption; using 192-bit for decryption with a 128-bit encrypted message will not work.
Return: You will get the decrypted Text (readable text) as String.
Usage in Blueprints
Encrypt String (AES)
- Category:
AESCryptor
- Description: Encrypts a given text using AES encryption.
- Inputs:
- PlainText (String): The text to encrypt.
- Key (String): The encryption key. The length must match the key size (16 bytes for 128-bit, 24 bytes for 192-bit, and 32 bytes for 256-bit).
- InitializationVector (String): A 16-byte value for encryption initialization.
- KeySize (EAESKeySize Enum): Select the key size: 128-bit, 192-bit, or 256-bit.
- Outputs (return):
- EncryptedText (String): The resulting encrypted text as a hexadecimal string.
Decrypt String (AES)
- Category:
AESCryptor
- Description: Decrypts an AES-encrypted text.
- Inputs:
- EncryptedText (String): The hexadecimal string to decrypt.
- Key (String): The decryption key. Must match the key used for encryption.
- InitializationVector (String): A 16-byte value used during decryption.
- KeySize (EAESKeySize Enum): Select the key size: 128-bit, 192-bit, or 256-bit.
- Outputs (return):
- DecryptedText (String): The decrypted original text.
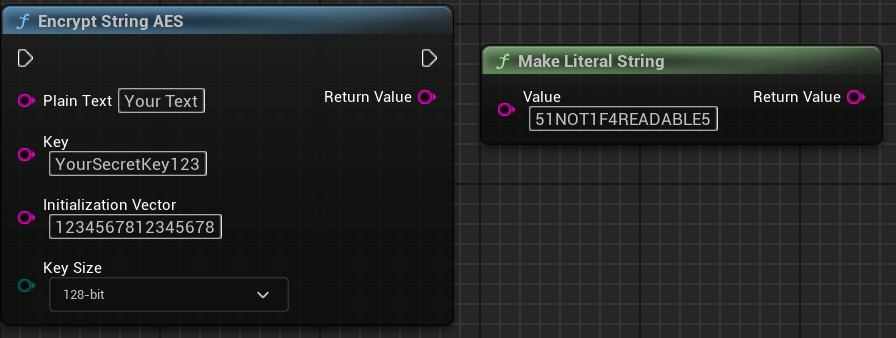
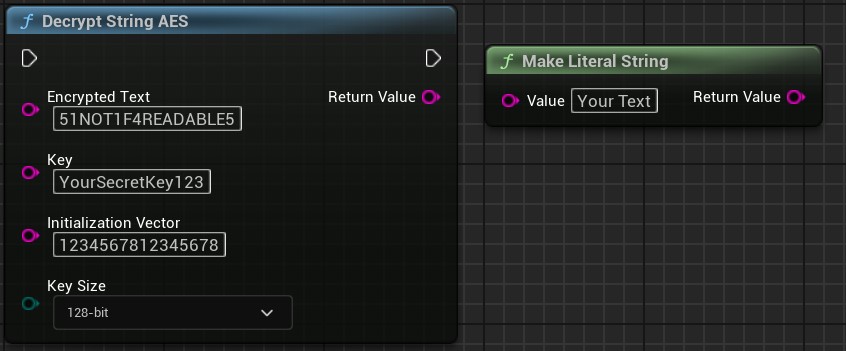
Blueprint Example
- Drag the Encrypt String (AES) node into a Blueprint.
- Set the
PlainText
to “Hello, Unreal Engine!”. - Provide a
Key
(e.g., “MySecretKey123456”). Make sure that your Key has the correct length (128-bit = 16 Characters) - Set the
InitializationVector
(e.g., “MyInitVector1234”). - Select
KeySize
to 128-bit. - Connect the output
EncryptedText
to a Print String node to display the encrypted message.
to decrypt:
- Drag the Decrypt String (AES) node into the same Blueprint.
- Use the output from the encryption as the
EncryptedText
input. - Ensure
Key
andInitializationVector
match those used during encryption. - Connect the output
DecryptedText
to another Print String node.
Usage in C++
EncryptStringAES
- Function:
FEncryptionHelper::EncryptStringAES
- Inputs:
FString PlainText
: The text to encrypt.FString Key
: The encryption key.FString InitializationVector
: The initialization vector.int32 KeySize
: The size of the key (128, 192, or 256).
- Output:
FString
– The encrypted string as a hexadecimal string.
DecryptStringAES
- Function:
FDecryptionHelper::DecryptStringAES
- Inputs:
FString EncryptedText
: The text to decrypt.FString Key
: The decryption key.FString InitializationVector
: The initialization vector.int32 KeySize
: The size of the key (128, 192, or 256).
- Output:
FString
– The decrypted string.
#include "EncryptionHelper.h"
#include "DecryptionHelper.h"
void MyEncryptionExample()
{
FString PlainText = TEXT("Hello, Unreal Engine!");
FString Key = TEXT("MySecretKey123456");
FString InitializationVector = TEXT("MyInitVector1234");
int32 KeySize = 128;
// Encrypt the text
FString EncryptedText = FEncryptionHelper::EncryptStringAES(PlainText, Key, InitializationVector, KeySize);
UE_LOG(LogTemp, Log, TEXT("Encrypted: %s"), *EncryptedText);
// Decrypt the text
FString DecryptedText = FDecryptionHelper::DecryptStringAES(EncryptedText, Key, InitializationVector, KeySize);
UE_LOG(LogTemp, Log, TEXT("Decrypted: %s"), *DecryptedText);
// Validate
if (DecryptedText == PlainText)
{
UE_LOG(LogTemp, Log, TEXT("Encryption and Decryption successful!"));
}
else
{
UE_LOG(LogTemp, Error, TEXT("Decryption failed. Original: '%s', Decrypted: '%s'"), *PlainText, *DecryptedText);
}
}
Troubleshooting & Common Issues
Invalid Key Length: Ensure the key length matches the selected key size (128-bit = 16 bytes, 192-bit = 24 bytes, 256-bit = 32 bytes).
Performance on Mobile: If you experience performance issues on mobile devices, consider using smaller key sizes (e.g., 128-bit) for faster processing times.
Initialization Vector (IV) Issues: The IV must always be exactly 16 bytes. If it’s not, padding or truncation errors may occur.
Decryption Errors: Ensure that the Key
, InitializationVector
, and KeySize
match those used during encryption.
Demonstration Content
You can find a demonstration map in the Unreal Engine’s AESCryptor Plugin Folder. Just go to “/All/Plugins/AESCrypter Content/Maps/” and load the Map. Hit “Play” and try it out by using the Demo UI which helps you to understand the plugin.
If you don’t see a “/Plugins/” Folder, go to your Content Drawer and click on the topper right corner on “Settings“. Activate “Show Plugin Content“.
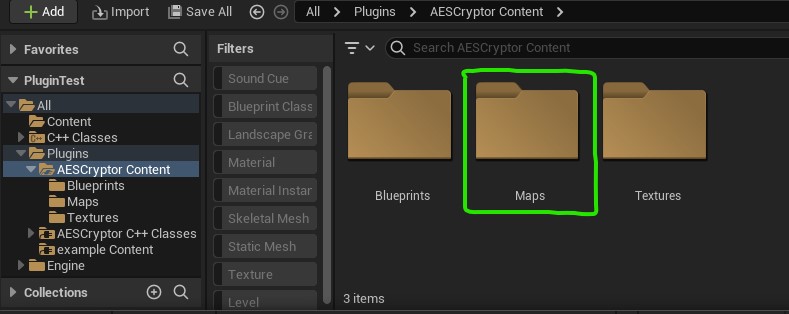
Roadmap
Initial Release.
- Initial release with AES-128, AES-192, and AES-256 encryption.
- Full Blueprint and C++ integration.
- Example project included for quick setup.
No entries.