Create INT and BOOL Matrices in Unreal Engine 5
Use DataMatrix in Unreal Engine 5 to create your own matrix variable. Whether 2D, 3D, or otherwise, store your BOOL or INT variables as a matrix.
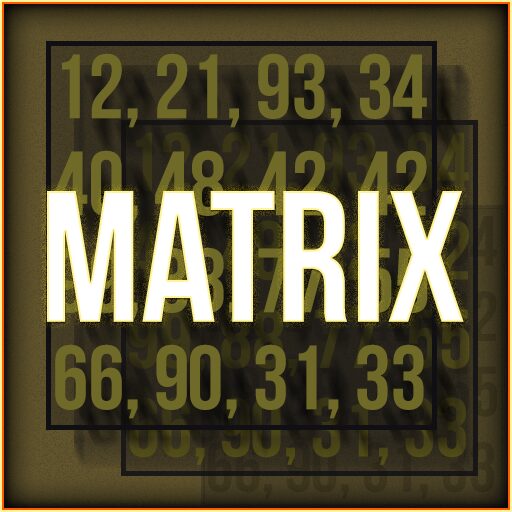
Description
The DataMatrix plugin for Unreal Engine enables the efficient management and processing of multidimensional matrices (int32
and bool
). It offers comprehensive support for Blueprints, C++, and DataTables, as well as multi-threading features for performance optimization with large data sets.
- Simple Matrices in Unreal Engine 5
- For BOOL and INT Variables
- C++-Integration, usable with C++
- 1-, 2-, 3-, 4-D Matrices and more
- Easily usable with Blueprints
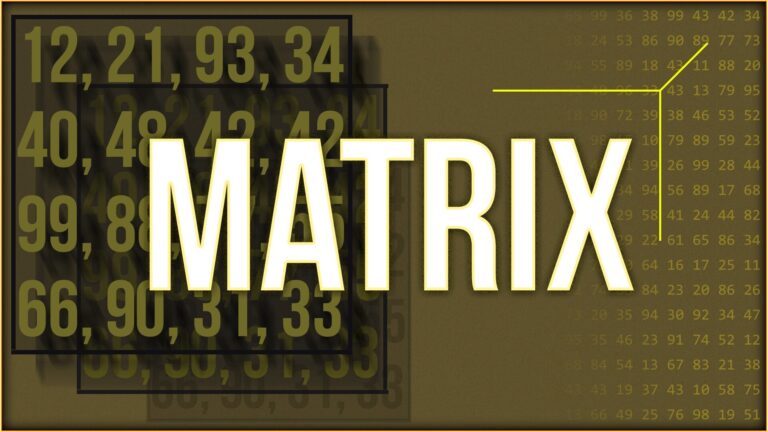
DataMatrix: Showcase
Use multidimensional matrices in Unreal Engine directly through Blueprint nodes or C++ functions. The DataMatrix plugin is ideal for projects on Windows, macOS, and Linux, offering efficient management of large data sets and easy integration. With support for DataTables and multi-threading, DataMatrix enables flexible and high-performance data structures. Thanks to comprehensive documentation and user-friendly Blueprints, getting started is effortless for both beginners and experienced developers.
Documentation & News
Table of Contents
Installation and Setup (for Source)
- Regenerate project files: Right-click on the
.uproject
file and select “Generate Visual Studio project files” (or the corresponding tool for your IDE). - Compile project: Open your project in Unreal Engine. The editor should automatically detect and compile the plugin.
- Enable plugin: Go to Settings > Plugins, search for “DataMatrix”, and ensure it is enabled.
- Enable plugin: Go to Edit > Plugins, search for “DataMatrix”, and ensure it is enabled.
YourProject/
└── Plugins/
└── DataMatrix/
File Structure
FDataMatrixInt
FDataMatrixInt
is a USTRUCT
that represents a multidimensional matrix for int32
values.
USTRUCT(BlueprintType)
struct DATAMATRIX_API FDataMatrixInt
{
GENERATED_BODY()
public:
UPROPERTY(BlueprintReadWrite, Category = "DataMatrix")
TArray IntMatrix;
UPROPERTY(BlueprintReadWrite, Category = "DataMatrix")
TArray Dimensions;
};
FDataMatrixInt
FDataMatrixBool
is a USTRUCT
that represents a multidimensional matrix for bool
values.
USTRUCT(BlueprintType)
struct DATAMATRIX_API FDataMatrixBool
{
GENERATED_BODY()
public:
UPROPERTY(BlueprintReadWrite, Category = "DataMatrix")
TArray BoolMatrix;
UPROPERTY(BlueprintReadWrite, Category = "DataMatrix")
TArray Dimensions;
};
Usage in Blueprints
Initialization of the Matrix
Synchronized Initialization
- InitializeMatrixInt / InitializeMatrixBool
-
- Description: Initializes an
FDataMatrixInt
orFDataMatrixBool
structure with the specified dimensions. - Inputs:
- Matrix (Reference): The matrix to be initialized.
- In Dimensions (Array of int32): The dimensions of the matrix, e.g.,
[2, 2]
for a 2×2 matrix.
- Example:
- Create a variable
MyMatrix
of typeFDataMatrixInt
. - Use the
InitializeMatrixInt
node and connectMyMatrix
to theMatrix
pin. - Create a
Make Array
with[2, 2]
and connect it toIn Dimensions
.
- Create a variable
- Description: Initializes an
Asynchronous Initialization with Multi-Threading
- InitializeMatrixIntAsync / InitializeMatrixBoolAsync
-
- Description: Initializes an
FDataMatrixInt
orFDataMatrixBool
structure asynchronously and invokes a callback. - Inputs:
- Matrix: The matrix to be initialized.
- In Dimensions (Array of int32): The dimensions of the matrix.
- On Completed (Delegate): The event that is called after initialization.
- Example:
- Create a variable
MyMatrix
of typeFDataMatrixInt
. - Use the
InitializeMatrixIntAsync
node and connectMyMatrix
,[2, 2]
, and a custom eventOnInitializeMatrixIntCompleted
.
- Create a variable
- Description: Initializes an
Setting and Retrieving Values
SetValueInt / SetValueBool
- Description: Sets a value (
int32
orbool
) at the specified indices. - Inputs:
- Matrix (Reference): The target matrix.
- Indices (Array of int32): The position, e.g.,
[0, 1]
. - Value: The value to set, e.g.,
42
orTrue
.
- Example:
- Use
SetValueInt
, connectMyMatrix
,[0, 1]
, and the value42
.
- Use
GetValueInt / GetValueBool
- Description: Retrieves a value (
int32
orbool
) from the specified indices. - Inputs:
- Matrix: The source matrix.
- Indices (Array of int32): The position, e.g.,
[0, 1]
.
- Output:
- Return Value: The retrieved value, e.g.,
42
orTrue
.
- Return Value: The retrieved value, e.g.,
- Example:
- Use
GetValueInt
, connectMyMatrix
and[0, 1]
, and connect the return value to aPrint String
.
- Use
Example: 2x2 and 2x2x2 Matrices
2×2 Matrix
- Initialization:
- Dimensions:
[2, 2]
- Dimensions:
- Setting Values:
[0,0] = 1
[0,1] = 2
[1,0] = 3
[1,1] = 4
- Retrieving Values:
- Retrieve and display values from
[0,0]
to[1,1]
.
- Retrieve and display values from
2x2x2 Matrix
- Initialization:
- Dimensions:
[2, 2, 2]
(Layer, Rows, Columns)
- Dimensions:
- Setting Values:
[0,0,0] = 1
[0,0,1] = 2
[0,1,0] = 3
[0,1,1] = 4
[1,0,0] = 5
[1,0,1] = 6
[1,1,0] = 7
[1,1,1] = 8
- Retrieving Values:
- Retrieve and display values from
[0,0,0]
to[1,1,1]
.
- Retrieve and display values from
[Layer, Row, Column]
are relevant, e.g. [1,1,1]
is the second layer, second row, second column.
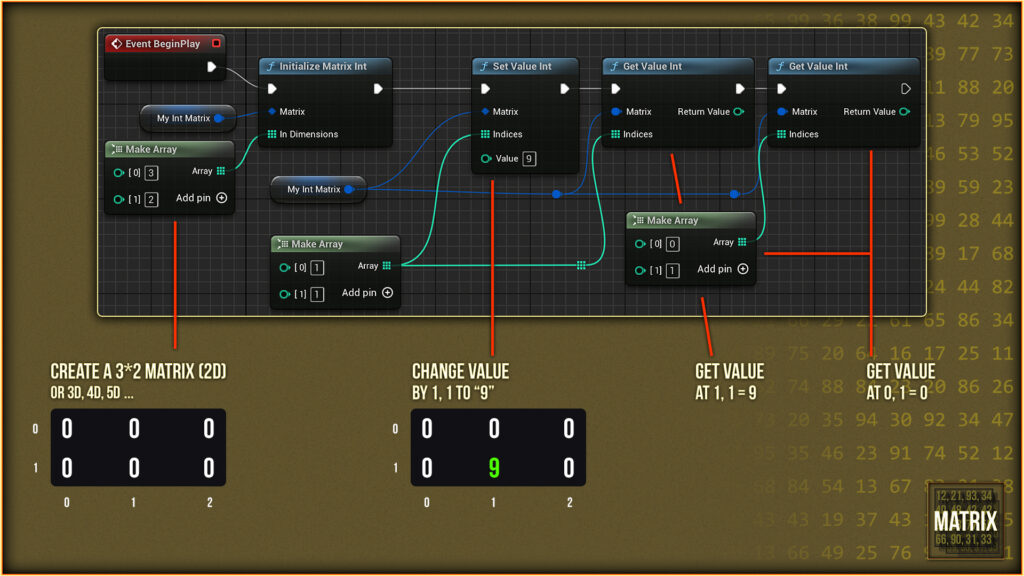
Usage in C++
Initialization of the Matrix
Synchronized Initialization
1. Inlcude Header:
#include "DataMatrixFunctionLibrary.h"
2. Declare and Initialize Matrix:
FDataMatrixInt MyMatrix;
TArray Dimensions = {2, 2};
UDataMatrixFunctionLibrary::InitializeMatrixInt(MyMatrix, Dimensions);
Asynchronous Initialization with Callbacks
1. Create Callback Function:
UCLASS()
class YOURPROJECT_API AYourActor : public AActor
{
GENERATED_BODY()
public:
virtual void BeginPlay() override;
UFUNCTION()
void OnInitializeMatrixIntCompleted(FDataMatrixInt Matrix);
};
2.Implementation of the Callback Function
void AYourActor::OnInitializeMatrixIntCompleted(FDataMatrixInt Matrix)
{
TArray Indices = {1, 0};
UDataMatrixFunctionLibrary::SetValueInt(Matrix, Indices, 42);
int32 Value = UDataMatrixFunctionLibrary::GetValueInt(Matrix, Indices);
UE_LOG(LogTemp, Log, TEXT("Wert an Position [1,0]: %d"), Value);
}
3. Start Async Init
void AYourActor::BeginPlay()
{
Super::BeginPlay();
FDataMatrixInt MyMatrix;
TArray Dimensions = {2, 2};
UDataMatrixFunctionLibrary::InitializeMatrixIntAsync(MyMatrix, Dimensions, FOnInitializeMatrixIntCompleted::CreateUObject(this, &AYourActor::OnInitializeMatrixIntCompleted));
}
Setting and Retrieving Values
Set a Value
TArray Indices = {1, 0};
UDataMatrixFunctionLibrary::SetValueInt(MyMatrix, Indices, 42);
Get a Value
int32 Value = UDataMatrixFunctionLibrary::GetValueInt(MyMatrix, Indices);
UE_LOG(LogTemp, Log, TEXT("Wert an Position [1,0]: %d"), Value);
Example: 2x2 and 2x2x2 Matrices
2×2 Matrix
1. Initialization:
FDataMatrixInt MyMatrix;
TArray Dimensions = {2, 2};
UDataMatrixFunctionLibrary::InitializeMatrixInt(MyMatrix, Dimensions);
2. Set a value
UDataMatrixFunctionLibrary::SetValueInt(MyMatrix, {0, 0}, 1);
UDataMatrixFunctionLibrary::SetValueInt(MyMatrix, {0, 1}, 2);
UDataMatrixFunctionLibrary::SetValueInt(MyMatrix, {1, 0}, 3);
UDataMatrixFunctionLibrary::SetValueInt(MyMatrix, {1, 1}, 4);
3. Get a Value
int32 Value00 = UDataMatrixFunctionLibrary::GetValueInt(MyMatrix, {0, 0});
int32 Value01 = UDataMatrixFunctionLibrary::GetValueInt(MyMatrix, {0, 1});
int32 Value10 = UDataMatrixFunctionLibrary::GetValueInt(MyMatrix, {1, 0});
int32 Value11 = UDataMatrixFunctionLibrary::GetValueInt(MyMatrix, {1, 1});
UE_LOG(LogTemp, Log, TEXT("Wert [0,0]: %d"), Value00);
UE_LOG(LogTemp, Log, TEXT("Wert [0,1]: %d"), Value01);
UE_LOG(LogTemp, Log, TEXT("Wert [1,0]: %d"), Value10);
UE_LOG(LogTemp, Log, TEXT("Wert [1,1]: %d"), Value11);
2x2x2 Matrix
1. Initialization:
FDataMatrixInt My3DMatrix;
TArray Dimensions3D = {2, 2, 2};
UDataMatrixFunctionLibrary::InitializeMatrixInt(My3DMatrix, Dimensions3D);
2. Set a value
UDataMatrixFunctionLibrary::SetValueInt(My3DMatrix, {0, 0, 0}, 1);
UDataMatrixFunctionLibrary::SetValueInt(My3DMatrix, {0, 0, 1}, 2);
UDataMatrixFunctionLibrary::SetValueInt(My3DMatrix, {0, 1, 0}, 3);
UDataMatrixFunctionLibrary::SetValueInt(My3DMatrix, {0, 1, 1}, 4);
UDataMatrixFunctionLibrary::SetValueInt(My3DMatrix, {1, 0, 0}, 5);
UDataMatrixFunctionLibrary::SetValueInt(My3DMatrix, {1, 0, 1}, 6);
UDataMatrixFunctionLibrary::SetValueInt(My3DMatrix, {1, 1, 0}, 7);
UDataMatrixFunctionLibrary::SetValueInt(My3DMatrix, {1, 1, 1}, 8);
3. Get a Value
UE_LOG(LogTemp, Log, TEXT("Wert [0,0,0]: %d"), UDataMatrixFunctionLibrary::GetValueInt(My3DMatrix, {0,0,0}));
UE_LOG(LogTemp, Log, TEXT("Wert [0,0,1]: %d"), UDataMatrixFunctionLibrary::GetValueInt(My3DMatrix, {0,0,1}));
// ... bis [1,1,1]
Usage with DataTables
Creating a DataTable
- 1. Choose Structure:
- Create a structure compatible with
FDataMatrixInt
orFDataMatrixBool
, e.g.,:
- Create a structure compatible with
USTRUCT(BlueprintType)
struct DATAMATRIX_API FDataMatrixIntStruct
{
GENERATED_BODY()
public:
UPROPERTY(BlueprintReadWrite, Category = "DataMatrix")
TArray Dimensions;
UPROPERTY(BlueprintReadWrite, Category = "DataMatrix")
TArray Data;
};
- 2. Create DataTable:
- Right-click in the Content Browser and select Miscellaneous > DataTable.
- Select the created structure (
FDataMatrixIntStruct
).
- 3. Name and Edit DataTable:
- Name the DataTable, e.g.,
DT_MyDataMatrixInt
. - Add new rows:
- Dimensions:
[2, 2]
for a 2×2 matrix. - Data:
[1, 2, 3, 4]
for the values.
- Dimensions:
- Name the DataTable, e.g.,
Initialize the Matrix from a DataTable
- Retrieve DataTable Row:
- Use the node “Get Data Table Row”.
- Select the DataTable
DT_MyDataMatrixInt
. - Enter the row name, e.g.,
Row1
.
- Break Structure:
- Use “Break FDataMatrixIntStruct” to access Dimensions and Data.
- Initialize Matrix:
- Use the node “InitializeMatrixWithDataInt”.
- Inputs:
- Matrix: Your matrix variable (
MyMatrix
). - In Dimensions: Connect to
Dimensions
from the DataTable. - In Data: Connect to
Data
from the DataTable.
- Matrix: Your matrix variable (
Explanation of the Blueprint Nodes
- Description: Initializes an
FDataMatrixInt
structure with the specified dimensions. - Inputs:
- Matrix (Reference): The matrix to initialize.
- In Dimensions (Array of int32): The dimensions of the matrix.
- Output: None.
- Description: Initializes an
FDataMatrixInt
structure with dimensions and predefined data. - Inputs:
- Matrix (Reference): The matrix to initialize.
- In Dimensions (Array of int32): The dimensions of the matrix.
- In Data (Array of int32): The data for the matrix.
- Output: None.
- Description: Sets an
int32
value at the specified indices. - Inputs:
- Matrix (Reference): The matrix where the value should be set.
- Indices (Array of int32): The indices of the position.
- Value (int32): The value to set.
- Output: None.
- Description: Retrieves an
int32
value from the specified indices. - Inputs:
- Matrix: The matrix from which the value should be retrieved.
- Indices (Array of int32): The indices of the position.
- Output:
- Return Value (int32): The retrieved value.
- Description: Changes the dimensions of an existing
FDataMatrixInt
structure. - Inputs:
- Matrix (Reference): The matrix to be resized.
- New Dimensions (Array of int32): The new dimensions.
- Output: None.
- Description: Clears the matrix data and dimensions of an
FDataMatrixInt
structure. - Inputs:
- Matrix (Reference): The matrix to be cleared.
- Output: None.
- Description: Returns the dimensions of an
FDataMatrixInt
structure. - Inputs:
- Matrix: The matrix whose dimensions are being queried.
- Output:
- Return Value (Array of int32): The dimensions of the matrix.
- Description: Returns the dimensions of an
FDataMatrixInt
structure as a comma-separated string. - Inputs:
- Matrix: The matrix whose dimensions are being queried.
- Output:
- Return Value (String): The dimensions as a string, e.g.,
"2,2"
.
- Return Value (String): The dimensions as a string, e.g.,
- Description: Asynchronously initializes an
FDataMatrixInt
structure with the specified dimensions and triggers a callback when the initialization is complete. - Inputs:
- Matrix: The matrix to be initialized.
- In Dimensions (Array of int32): The dimensions of the matrix.
- On Completed (Delegate): The event triggered after initialization.
- Output: None.
FDataMatrixInt
versions but replace int32
with bool
.
Troubleshooting & Common Issues
DECLARE_DYNAMIC_DELEGATE_OneParam
and passed as const&
in functions.
TArray
: Ensure that TArray
assignments are correct and do not violate const
qualifiers. Use mutable
in lambda functions when necessary.
mutable
to allow modifications to captured copies.
Roadmap
Initial Release.
- Initial release
BOOL Matrix
INT Matrix
Get, Set, Init, Clear, Resize
1D, 2D, 3D, 4D, 5D … Matrices
No Entries.